Airdrop NFTs
Engine makes it effortless for any developer to airdrop NFTs at scale. You sponsor the gas so your users only need a wallet address!
This guide references Polygon Mumbai testnet and NextJS but is applicable for any EVM chain and full-stack framework.
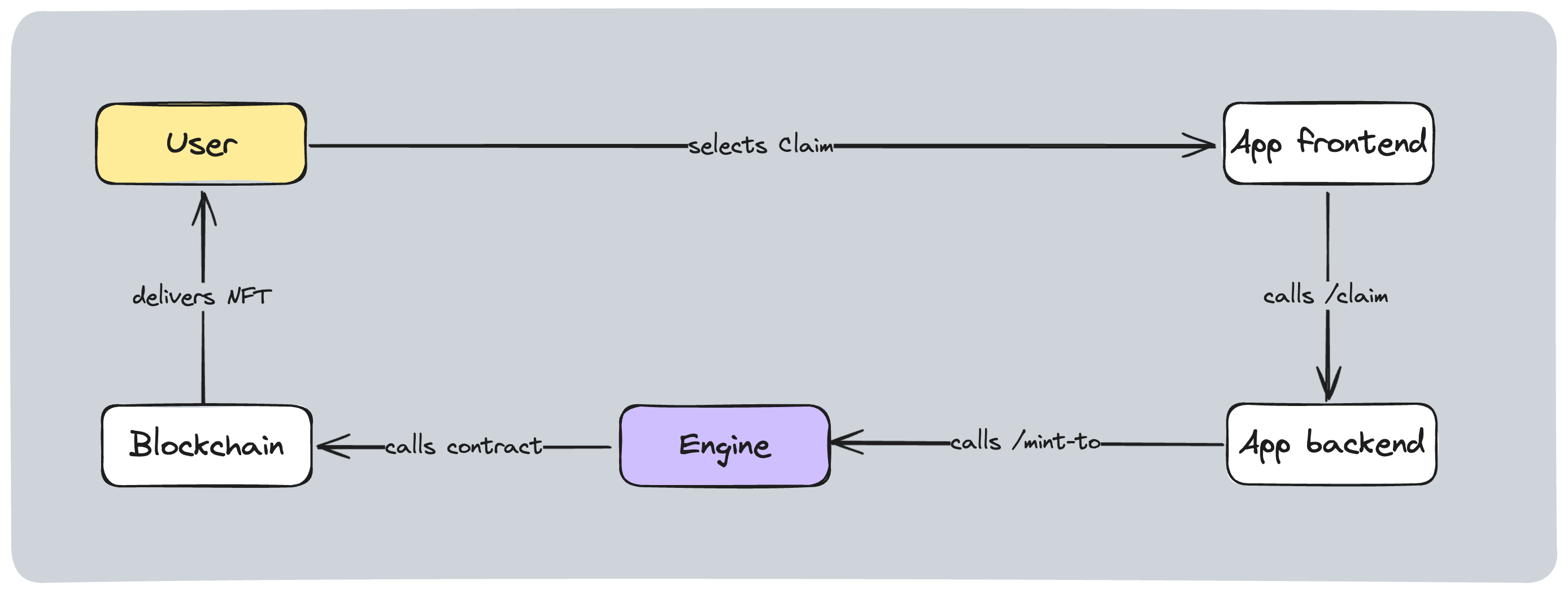
Prerequisitesβ
- An Engine instance
- A backend wallet with MATIC on Mumbai
- A deployed NFT contract that can be claimed by the backend wallet
- A client ID and secret key from the API Keys page
Frontend: Add Connect Wallet and Claim buttonsβ
Use <ConnectWallet>
to prompt the user for their wallet. The Claim button calls POST /api/claim
.
export default function Home() {
return (
<ThirdwebProvider activeChain="mumbai" clientId="<thirdweb_client_id>">
<ClaimPage />
</ThirdwebProvider>
);
}
function ClaimPage() {
const userWalletAddress = useAddress();
const onClick = async () => {
await fetch("/api/claim", {
method: "POST",
body: JSON.stringify({ userWalletAddress }),
});
alert(`π A reward has been sent to your wallet: ${userWalletAddress}`);
};
return (
<main>
<h2>Thank you for being a superfan! β€οΈ</h2>
<ConnectWallet />
{userWalletAddress && <button onClick={onClick}>Claim my reward</button>}
</main>
);
}
Replace <thirdweb_client_id>
.
Backend: Call Engine to mint an NFTβ
POST /api/claim
calls Engine to mint an NFT to the user's wallet.
export async function POST(request: Request) {
const { userWalletAddress } = await request.json();
await fetch(
"<engine_url>/contract/mumbai/<nft_contract_address>/erc1155/mint-to",
{
method: "POST",
headers: {
"Content-Type": "application/json",
Authorization: "Bearer <thirdweb_secret_key>",
"x-backend-wallet-address": "<backend_wallet_address>",
},
body: JSON.stringify({
receiver: userWalletAddress,
metadataWithSupply: {
metadata: {
name: "Acme Inc. Superfan",
description: "Created with thirdweb Engine",
image:
"ipfs://QmciR3WLJsf2BgzTSjbG5zCxsrEQ8PqsHK7JWGWsDSNo46/nft.png",
},
supply: "1",
},
}),
},
);
return NextResponse.json({ message: "Success!" });
}
Try it out!β
Hereβs what the user flow looks like.
The app prompts the user to connect their wallet.
A user presses claim.
They'll receive the NFT in their wallet shortly!
Full code exampleβ
The code above is simplified for readability. View the full source code: